How to Perform Sentiment Analysis With TextBlob
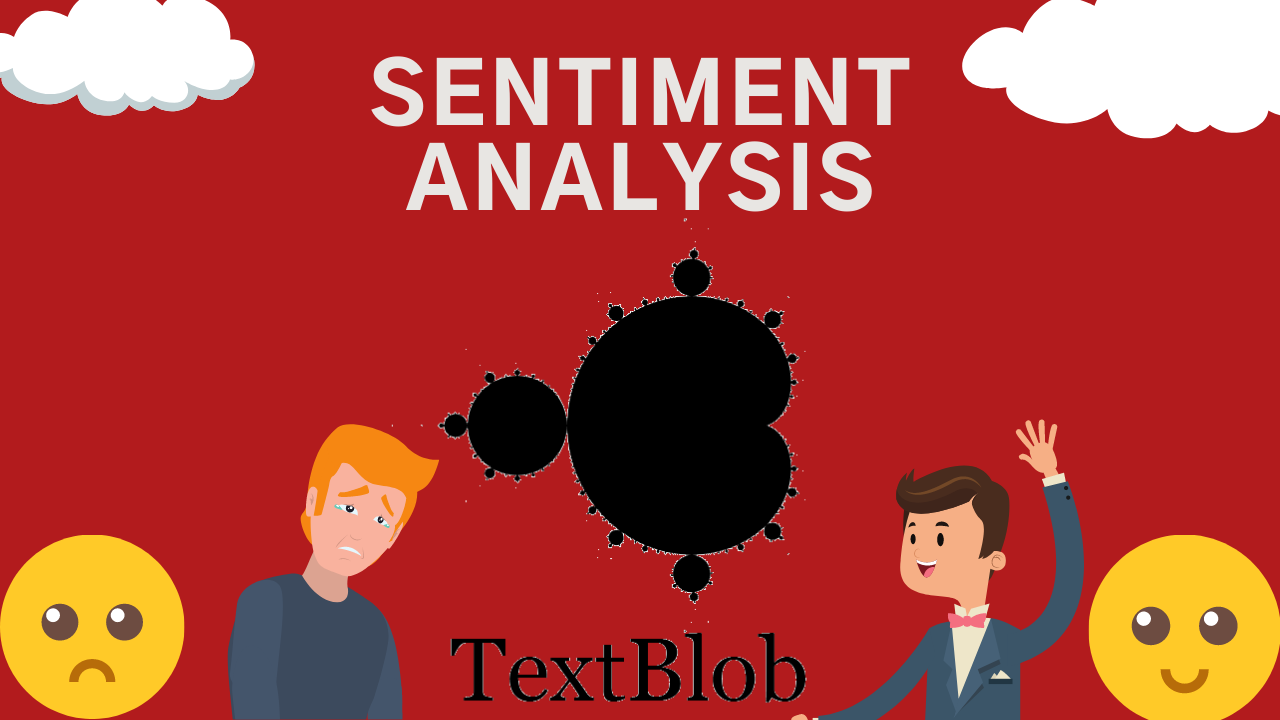
In this article, we'll discuss how to use TextBlob to perform sentiment analysis. TextBlob is an easy-to-use open source Python package that makes it easy to perform fundamental NLP tasks like tokenization, noun phrase extraction, classification and more. TextBlob is built on top of NLTK – a renowned NLP Python library. And, in recent years, it has been gaining popularity, with currently 7.7K stars on GitHub.
Sentiment Analysis With TextBlob
First off, let's install TextBlob.
pip install textblob
Now, import a class called TextBlob.
from textblob import TextBlob
From here, we can use the TextBlob class to create an object for the text we want to classify.
blob = TextBlob("Performing sentiment analysis with TextBlob is fun and easy!")
We can now perform many different tasks with our newly instantiated object. Call the object's "sentiment" method to perform sentiment analysis.
result = blob.sentiment
print(result)
Result: Sentiment(polarity=0.4208333333333334, subjectivity=0.5166666666666667)
The result is a dataclass object with two variables: polarity and subjectivity. Polarity represents the sentiment and is a float between -1 and 1, where higher values indicate positive sentiment. Subjectivity represents how subjective and is a float between 0 and 1. A subjectivity score of 1 means the text is very subjective. Likewise, a subjectivity score of 0 means the text is objective.
We can isolate one scores by accessing the desired variable.
result = output.sentiment
print(result)
Result: 0.4208333333333334
This score represents that the text has positive sentiment, which makes sense. Below is an example of text with negative sentiment.
blob = TextBlob("I hate NLP technology that's hard to understand")
result = blob.sentiment
print(result.polarity)
Result: -0.5822916666666667
Subjectivity vs Objectivity
Here are two examples that help clarify what it means for text to be subjective vs objective.
Subjective
blob = TextBlob("I kind of think TextBlob is very good")
result = blob.sentiment
print(result.subjectivity)
Result: 0.8400
Objective
blob = TextBlob("TextBlob has 7700 stars on GitHub")
result = blob.sentiment
print(result.subjectivity)
Result: 0.0
Speed
Some of you may be wondering, "what's the point of using traditional NLP technologies when we can implement deep learning models, like Transformer models?" Well, I think the clearest answer to that is the computational requirements of each method. Transformer models typically perform better than traditional approaches on text classification problems. But, depending on your computational demands and hardware limitations, you may want to use TextBlob to reduce your computational requirements.
Note: This is just a quick example -- not a scientifically valid experiment. For example, multiple different inputs should be used.
Here is an example of how long it takes to perform 1000 sentiment classification with TextBlob. The experiment was performed on a free Google Colab CPU instance.
start = time.time()
for i in range(0, 1000):
output = TextBlob("I just had so much fun learning how to use TextBlob to perform sentiment analysis")
output.sentiment
end = time.time()
print(end-start)
result: 0.20745253562927246(seconds)
So, it only took 0.21 seconds to classify 1000 examples!
Conclusion
And that's it! You just learned how to perform sentiment analysis with TextBlob. If you want to achieve higher accuracy, then I suggest you check out this video I created on how to implement a Transformer model for sentiment analysis.
Misc
Be sure to subscribe to our newsletter and subscribe to our YouTube channel for more content like this.
Stay happy everyone!
Book a Call
We may be able to help you or your company with your next NLP project. Feel free to book a free 15 minute call with us.